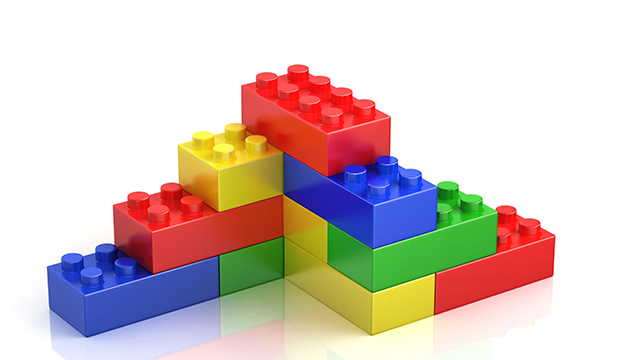
Build your nodeJS Application in a modular way
Now a days almost all web services or integrations are done on top of nodeJS run-time. NodeJS is a flexible platform with lot of community support. Even it is possible to create documents like xlsx, docx or pdf directly from nodeJS. All major cloud platforms uses nodeJS as their level 1 language.
Modularity
NodeJS by design achieves the modularity by using the node_modules structure. All the required modules are stored in node_modules directory and we can invoke the modules any where in our code.
But are we using this modularity in our application code. Most of the application I saw contains a lib folder, i which we store all js files . This js files are imported in the required areas using relative paths.
const db = require("../db/")
const logging = require ("../../logging")
The main problem with this kind of approach is that when we change the path of a service file the path to the db should change. Also the format is not readable. We will be confused with the file’s authenticity.
The Solution
A much better approach is to design our application as modules, such as db , logging, error etc . Lets say your application name is cms, then it is much easier to represent the module using scope
require("@cms/db")
You can develop the modules separately and publish in to any NPM servers ( public / private ) and use it as like any other module.
If your application needs the logging module
npm i --save @cms/logging
If you don’t want to split your application in to bits and pieces, there is another approach.
A more better way
Keep the modules that you want inside a separate folder. Lets say “@cms”. Use separate folder for each module and let the modules have a separate package.json. This way it will become a valid node module.
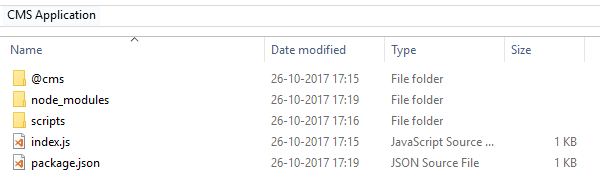
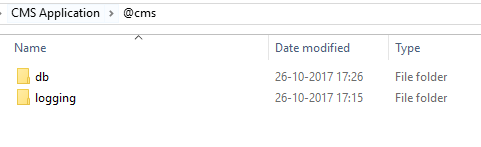

The package json for the modules will look like this
{
"name": "@cms/db",
"version": "1.0.1",
"description": "db module for CMS Application",
"main": "index.js",
"dependencies":{
"mysql" : "latest"
}
}
Once the modules are ready now its time to do some scripting. Add the install.js in the “scripts” folder.
let fs = require('fs')
console.log('Creating symlinks ...')
if (fs.existsSync('node_modules/@cms')) {
console.log('link exists already ')
} else {
let source = '../@cms'
console.log(`creating link for ${source}`)
fs.symlinkSync(source, 'node_modules/@cms', 'junction')
console.log('done')
}
Add this script to your main package.json.
{
"name": "CMSApplication",
"version": "1.0.1",
"description": "Sample CMS Application",
"main": "index.js",
"scripts": {
"install": "node scripts/install.js",
"start": "node index.js"
},
"dependencies":{
"express" : "latest"
}
}
The script will be executed every time you do npm install. So once all other node modules defined in the dependencies are installed, it will create a link from the @cms folder outside to the @cms folder inside node_modules. So any changes you make to the outer @cms folder will be reflected to the folder inside node_modules.

You can see that the symlinks are installed for @cms. This is not a shortcut file rather that hard links created using “ln” in linux.
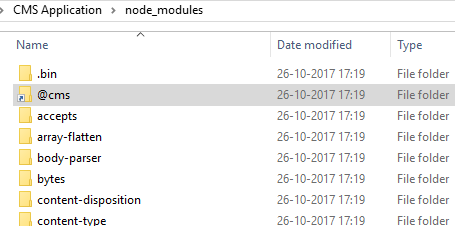
Inside @cms you can see our modules which is defined in the outer @cms folder
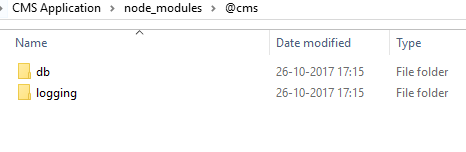
This way you can achieve modularity. “@cms” folder is part of your source code. You can then import the required modules in the normal way.
This approach helps me in making the application more modular and extensible. Let me know your thoughts on this.
Do you Google App Engine allows to to create symlinks?
This post is now obsolute. Now npm supports dependencies with file:// protocol, which is automatically linked inside the node_modules.